C Programming
Complete C language
What is Programming.....?
- Computer programming is a medium for us to communicate with computers. Just like we use some regional languages to communicate with each other . same with the use of programming we deliver our instruction to the computers.
What is C....?
C is a programming language.
C is the one of the oldest and finest programming language.
- C language Was developed by Dennis Ritchie at AT & T's bell labs, USA in 1972.
Uses of C
- C language is use to program wide variety of system some of the uses of are as follows.
- Major part of windows ,Linux and other operating system are written in C.
- C is used to write driver programs for devices like Tablet, printers etc.
- C language is used to program embedded systems where programs need to run faster in limited memory (Microwave, cameras etc.)
Variables
- A variable is a container which stores a 'value' .
- In kitchen, we have containers storing Rice, Dal, Sugar etc.
- Variable is an Entity whose value can be change.
- similarly variable in C store value of a Constant Example:
Rules for naming variable in C
First character must e an alphabet or underscore(_)
No comma, blank space are allowed
No special Symbol other than (_) allowed.
Variable names are cause sensitive
- We must create meaningful variable names in our programs. This enhance readability of our programs.
Constant
An Entity whose value doesn't change is called as constant.
Examples : - 1, 2,3,4,A and lot more are the examples of constant. As this is defined in our definition pretty well.
Types of constants
- Integer Constant š -1 , 5 ,10 ,23(It don't contain any fractional parts )
- Real Constant š -45.4, 6.7 , 7.0 (It my or may not contain fractional parts )
- Character constant š 'a' , '$' , 'g' (Must be enclosed in an single inverted comma)
Keywords
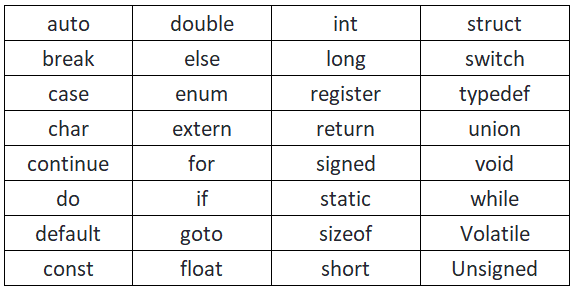
Our First C program
link :- Click here to get the practice note .
Basic Structure of a C Program
- Every program's execution starts from main() Function.
- All the Statement are terminated with a semicolon.
- Instructions are case- sensitive
- Instructions are executed in the same order in which they are written
Comments
- Single line comments : // this is a comments
- Multi-line Comments : /* This is a multi line comments */
Compilation and Execution

Library Functions
- %d for integers
- %f for real values
- %c for characters
link :-
Receiving input from the user
Syntax for using scanf:
scanf ("%d",&i)
Link:-
Practice set :-
- write a c program to calculate area of rectangle :
- using hard codded inputs
- Using input supplied by the user
calculate the area of the circle and modify the same program to calculate the volume of the cylinder gives its volume and height.
Link :- click here to get the solution of above problem.
- Write a program to covert a celsius (centigrade degrees temperature to Fahrenheit )
Link :- click here to get the solution of the above problem.
- write a program to calculate simple interest for a set of values representing principal , number of years and rate of interest.
Link :- click here to get the solution of the above problem.
Instruction and operators
Types of instructions
- Type declaration instruction
- Arithmetic Instruction
- Control instruction
Type declaration Instruction :
1.Type declaration instruction
- int a ;
- float b;
- Other variations :
- int i = 10; int j = i ; int a = 2
- int ji = a +j -i
- float b = a+3; float a = 1.1 => (here we got the error as we are trying to use it before defining it .)
- int a,b,c,d;
- a = b = c = d = 30 ; => (The value of a,b,c&d will be 30 each )
2. Arithmetic instructions
- int i = (3 * 2) + 1
- operands can be int/float etc.
- +,-,*,/ are arithmetic operators
- int b = 2 , c = 3;
- int z = b*c; (Legal)
- int z; b*c = z;(in legal not allowed )
- % => Modular division operator
- Return the reminder
- cannot be applied on the float
- sign is same as of numerator (-5%2 = -1 )
- 5% 2 = 1
- -5% 2 = -1
Note : -
- No operator is assumed to be present
- int i = ab =>Invalid
- int i = a*b =>valid
Type Conversion : -
- int and int => int
- int and float = > float
- float and float => float
- important
- 5/2 => 2 5.0/2 = 2.5
- 2/5 => 0 2.0/5 = 0.4
Note :-
- int a = 3.5; In this cause 3.5(float) will be demoted to 3(int) because a is not able to store floats.
- float a = 8 ; a will store 8.0 8=> 8.0 (promotion to the float)
Operator Precedence in C :-
3*x - 8y is (3x)-(8y) or 3(x-8y)..?
In C language simple mathematical rules like BODMAS , No longer applies.
The answer for the above Question is provided by operator precedence & associativity.
Operator precedence :-

Operator Associativity:-
- x * y / z => (x*y)/z
- x / y * z => (x/y)*z
- *,/ follow left to right associativity.
Link :- Click here to get the link of above practice code.
Control Instructions:
- Sequence control Instruction
- Decision Control instruction
- Loop control instruction
- Cause Control Instruction
Practice set:-
- Which of the following are invalid in C..?
- int a; b = a;
- int v = 3^3;
- char dt = '21 Dec 2020'
- What data type will 3.0/8 -2 Return ....?
- Answer :- double /float(because most of the compiler consider double to every decimal number it's consider as double until the data type are not defined )
- Write the program to check whether a number is divisible by 97 or not .
Link :- Click here to get the practice code of above problem.
- Explain step by step evaluation of 3*x/y-z+k
- Where x = 2 , y = 3 , z = 3 , k = 1
Link :- click here to get the above practice code of above problem.
- 3.0 + 1 will be :
- Integer
- Floating point number š(Answer)
- Character
Conditional Instruction:
Decision Making instruction in c :
- if else Statement
- The syntax of an if-else Statement in C look like
- if (Condition to be checked){
- statements -if condition is true ;
- }
- else {
- statement if the condition is true ;
- }
- else is optional not mandatory if the condition of if is false than it run
Relational operators in C

Note :- '=' is used for the assignment operator where '==' is used for the equality check
Logical operators:-

Usage of logical Operator :-
- && --> AND --> is true when both the conditions are true
- "1" and "0" is Evaluated as false.
- "0" and ''1" is Evaluated as false.
- "1" and "1" is Evaluated as True
- || --> OR --> is true when at least one of the condition is true
- "1" and "0" is Evaluated as True.
- "0" and ''0" is Evaluated as false.
- "1" and "1" is Evaluated as True
- ! --> return true if given false and false if given True.
- ! (3==3) --> Evaluates to false
- !(3 > 30) --> Evaluate to true.
- As the number of condition increases the level of indentation increases This reduces the readability logical operators to rescue in such cases
Else if Clause
Conditional operator:
- Syntax
- condition ? expression -if-true : expression if-false
Switch cause Control Instruction
switch cause is used when we have to make a choice between number of alternatives for a guven Variable.
Syntax
Switch (integer-expression)
{
case c1:
code;
case c2:
code;
case c3:
code;
}
Link:- Click here to get above practice code.
- 90-100 --> A
- 80-70 --> B
- 70-80 --> C
- 60-70 --> D
- <60 --> F
Important Note :-
- We can use switch- case statements even by writing cases in any order of our choice (not necessarily ascending)
- char values are allowed as they can be easily evaluated to an integer.
- A switch an occur with in another but in practice this is rarely done
Practice set :-
- What will be the output of this program
- Output :- I am 11 (because 1= is a assignment operator )
- Write a program to find at whether a student is pass or fail; if it required total 40% and at least 33% in each subject to pass. Assume 3 subject take marks input from the user .
- Calculate income tax paid by an employee to the government as per the slabs mentioned below
- income slab Tax
- 2.5L - 50L 5%
- 5.0L - 10.0L 20%
- above 10.0L 30%
- Note :- there is no tax below 2.5l Take income amount as an input from, the user
Link :- click here to get the solution of above practice problem.
- Write a program to find whether a year entered is a leap year or not. take year as an input from the user .
Link:- Click here to get the solution of above practice problem.
- write a program to determine the character entered by the user is lower cause or not.
Link :-click here to get the solution of the above problem.
- Write a program to find greatest of 4 number entered by the user.
Loop Control Instruction :-
Why loops
Types of Loops:-
While loop
Example:- Click here to get the example.
Note:- If the condition never become false, the while loop keeps getting executed. Such a loop is known as an infinite loop.
Quick Quiz :- Write a program to print natural number from 10 to 20 When initial loop counter i is initialized to 0.
- i++ => i is in increment operator
- i-- => i is decrement operator
- +++ operator does not exist
- += is the compound assignment operator
- just like -=,+= . /= & %=
do-while loop.
For Loops
The Break Statement in C language.
The Continue Statement in C.
Note:-
- Sometimes, the name of the variable might not indicate the Behaviour of the program.
- Break Statement Completely Exits the loop.
- Continue Statement skips the particular statements of the loop.
Practice set:-
- at least once.
- at least twice.
- at most once.
Project 1 : number guessing game
problem :-
We will Wrote a program that generate the random number Ask the player to guess it. If the player,s guess is higher than the actual number, the program display "Lower number please". Similarly if the user guess is higher than the program print "higher number please" .
when the player guesses the correct number, the program display the number of guesses the player use to arrive at the number .
Link :- Click here to get the link of the solution of this game.
Function And Recursion:-
Example and syntax of a function
Function prototype :-
Important points :-
- Execution of a C program start from main()
- A C program can have more than one function.
- Every Function gets called directly or indirectly from main()
- There are two types of function in c. lets talk about them.
Types of function
- Library Functions:- Commonly required functions grouped together in a library file on disk.
- User Defined Functions :-These are the function declared and defined by the user.
Why use Function.....?
- To avoid recreating the same logic again and again.
- To keep track what we are doing in the program.
- To test and check logic independence
Passing values to Function.
Recursion :-
Important notes:-
- Recursion is sometimes the most direct way to code an algorithm
- The condition which doesn't call the function any Further in a recursive function is called as the base function
- Sometimes, due to a mistake made by the programmer, recursive function in a memory error.
Practice set:-
Pointers

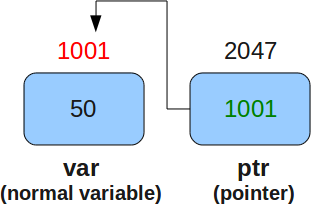
How to declare a pointer. ...?
Pointer to a pointer
Types of function call's :-
- Call by value => Sending the value of argument.
- call by reference. => Sending the address of argument.
Call by reference.
Practice set:-
Arrays:-
An array is a collection of similar elements.
one variable => Capable of storing multiple similar value.
Syntax:-
The syntax of declaring an array look like this.
int marks[90]: => integer array which have capacity of storing 90 int data.
int name[20]: => Character array or string. which have a capacity of storing 20 char data.
int percentile[90]: => Float array which have a capacity of storing 90 float data.
The value can now be assigned to marks array like this.
Accessing elements:-
Elements of an array can be accessed using.
Link:- Click here to get the program of implementation of an array.
Quick quiz :-
Write a program to accept marks of five students in an array and print them to the screen.
Link :- Click here to get the solution of above quiz.
Initialization of an Array :-
There are many others ways in which in which an array can be initialized.
Link:- Click here to see the more way of initialization of array.
Array in memory.
Consider this array
int arr[3] = {1, 2 ,3 } => 1 integer = 4 bytes
This will reserve 4*3 = 12 bytes in memory 4 bytes for each integer.
array always store in continuous form in the memory.
Pointer Arithmetic.
A pointer can be incremented to point to the next memory location of that data type.
Link:- Click here to get the example and implementation of pointer arthmatic.
- Addition of a number to a pointer.
- subtraction of a number from a pointer
- subtraction of one pointer from another
- comparison of two pointer variable.
Accessing Arrays using pointers.
Link:- Click here to get implementation of initialization of array using the pointer.
If ptr points to index 0 , ptr++ will points to index 1 & so on.
passing arrays to functions:-
Arrays can be passed to function like this
Link:- Click here to get the implementation of passing the arrays to functions.
Multidimensional Arrays.
An array can be of 2 dimension/3 dimension / n dimensions.
A 2 dimensional array can be defined as :
int arr[3][2] = { { 1, 4}
{7, 9}
{11, 22}};
We can access the elements of this array as arr[0][0], arr[0][1] & So on....!
Link:- Click here to get the link of the implementation of 2d array.
Quick quiz. :- Create a 2-d array by taking input from the user. Write a display function to print the content of 2d array on the screen.
Link:-
Practice set:-
1. Create an array of 10 number. verify using pointer arithmetic that (ptr +2) points to the third element where ptr is the pointer pointing to the first element of array.
Link :- Click here to get the solution of above practice problem.
2. If S[3] is a 1-D array of integers then *(s+3) refers to the third elements.
- True
- False
- Depends.
4. Repeat problem 3 for a general input provided by the user using scanf .
Link:- Click here to get the solution of above practice problem.
5. Write a program containing a function which reverse the array passed to it.
Link:- Click here ti get the solution of above practice problem.
6. write a program containing Functions Which counts the number of positive integer in the array?
Link:- Click here to get the solution of the above practice problem.
7. Create an array of size 3*10 containing multiplication table of the number 2,7 and 9 respectively.
Link:- Click here to get the link of the above practice problem.
8. Repeat problem 7 for a custom input given by the user.
link:- Click here to get the link of the above practice problem.
9. Create a three-Dimensional array and print the address of it's elements in increasing order.
Link:- Click here to get the link of above practice problem.
String:-
A string is a 1-D Character array terminated by a null ('\0')
('\0') = null character
Null character is used to store in a string termination Characters are stored in contiguous memory location
Initializing String :-
char s[] = {'D', 'E', 'E','P','A','K','\0'};
There is another shortcut for initializing string in C language :
char s[] = "HARRY"; => In this cause c add the null character automatically
Link :- Click here to get the program of The initialization of string.
String in a Memory:-
A string stored just like array in the memory as shown below
Quick Quiz. :- Create a string using " " and print its content using a loop
Link :- Click here to get the solution of quick quiz.
Printing String:-
A string can be printed character by character using printf and %c
but there is another convenient way to print the string in C language.
Link :- Click here to see the more convenient way to print the string.
Taking String input from the user:-
Note:
The string should be short enough to fit into the array
- Scanf cannot be used to input multi-word strings with spaces.
Gets() and puts()
gets() is a function which can be used to receive a multi- word strings.
Declaring a string using pointers:-
we can declare strings using pointers.
Note :-
Once a string is in initialized using char st[] = "Deepak", it cannot be re - initialized to something else.
A string defined using pointers can be reinitialized ptr = "Randeep"
Standard library functions for strings.
C provide a set of standard library functions for string manipulation.
Strlen()
This function is used to count the number of character in the string excluding the null('\0') character
strcpy()
This function is used to copy the content of second string into first string passed to it
char soure[] = "Deepak"
Char target[30];
strcpy(target, source); target now contain "Deepak"
strcat()
strcmp()
Practice set :-
1. Which of the following is used to appropriately read a multi-word string
- gets()
- puts()
- printf()
- scanf()
2. write a program to take string as an input from the user using %c and %s confirm that the string are equal .
Link:- Click here to get the solution of above practice problem.
3. write your own version of strlen function from <string.h>
Link:-Click here to get the solution of above practice problem.
4. Write a function slice() to slice a string, it should change the original string that it is now the sliced string . Take m and n as the start and ending position for slice.
Link:- Click here to get the solution of the above practice problem.
5. Write you own version of strcpy function from <string.h>
Link :- Click here to get the solution of the above practice problem.
6. Write a program to encrypt a string by adding 1 to the ascii value of the characters.
Link:- Click here to get the solution of the above practice problem.
7. Write a program to decrypt the string encrypted using encrypt function in problem 6.
Link:- Click here to get the link of above practice problem.
8. Write a program to count the Occurrence of a given character in a string.
Link:- Click here to get the solution of the above practice problem.
9. Write a program to check Whether a given character is present in a string or not.
Link :- Click here to get the solution of the above practice problem.
Structure :-
Arrays and strings => similar data (int , float char)
Structures can hold => dissimilar data
Syntax for creating Structure:-
A c Structure can be created as follows:-
Struct Employee {
int Code;
float Salary;
char name[10];
}
We can use this User defined data types as follows:
Link :- Click here to get the program of the implementation of the structure.
So a structure in C is a Collection of variables of different types under a single name.
Quick Quiz:- Write a program to store the details of # employees from user defined data. Use the Structure declared above.
Link:- Click here to get the solution of the above Quick quiz.
Why use Structure....?
We can create the data types in the Employee Structure but When the number of properties in a Structure increases, it becomes difficult for us to create data variables Without Structures. In a nut shell- Structure keep the data organized
- Structure make data management easy for the programmer.
Array of Structure :-
Just like an array of integers, an array of floats and an array of characters, we can create an array of Structure .
Struct employee facebook[100]; => An array of Structures
We Can access the data using:
facebook[0].code = 100;
facebook[1].code = 101;
Initializing Structures :-
Structures can also be initialized as follows:-
Link :- Click here to get the program of another way of initializing the structure.
Structure in memory:-
Structure are stored in contiguous memory locations For the Structure e1 of type Struct employee , memory layout looks like this:
In an array of Structure these employee instances are stored adjacent to each other.
Pointer to Structure :-
A pointer to Structures can be created as follows:
Struct employee*ptr;
ptr = &e;
Now we can print Structure elements
printf("%d", (*ptr).code);
Link :- Click here to get the implementation of pointer to the structure.
Arrow Operator :-
Instead of Writing (*ptr).code , we can use arrow operator to access structure properties as follow
(*ptr).code or ptr->code
here is known as the arrow operator
Passing Structure to a Function:-
A structure can be passed to a function just like another data types.
Void show(struct employee e); => Function prototypes
Quick Quiz :- Complete this show function to display the control of employee.
Link :- Click here to get the Solution of above quick quiz.
Type def keyword :-
We can use the typedef keyword to create an alias name for data types in c. typedef is more commonly used with Structures.
Struct complex {
float real; => Struct complex C1,C2 ; for defining complex numbers
float img;
}
type def struct complex{
float real; => complex no C1 , C2; for defining complex numbers
float img
} complexno;
Link:- Click here to get the practice problem of typedef keyword.
Practice set :-
1. Create a two dimensional Vector using Structure in C.
Link :- Click here to get the Solution of above practice problem.
2. Write a Function sumvector Which return the sum of two vector passed to it . The vector must be two dimensional.
Link :- Click here to get the solution of above practice problem.
3. Twenty integer are to be stored in the memory what would you prefer array or structure....?
Answer :- Array because all those 20 are integer.
4. Write the program yo illustrate the use of arrow operator -> in C language.
Link :- Click here to get the solution of the above practice problem.
5. Write a program with a structure repeating the complex number ...?
Link :- Click here to get the solution of the above practice problem .
6. Create an array of 5 complex numbers created in problem 5 an display them with the help of display function The value must be taken as an input from the user.
Link:- Click here to get the solution of the above practice problem.
7.Create a structure representing a bank account of a customer . what fields did you use and why...?
Link :- Click here to get the solution of the above practice problem.
8. Write a structure capable of storing data write a function to compare those dates.
Link :- Click here to get the solution of the above practice problem.
9. Solve problem 9 for time using typdef keywords...?
Link :- Click here to get the link of the above practice problem.
File I/O:-
The random Access memory is volatile and its content is lost once the program terminates. A C program can talk to the file by reading content from it and writing control to it.
A file is data stored in storage in a storage device. A C program can talk to the file by reading content from it and writing content to it .
FILE pointer :-
"FILE" is structure which needs to be created for opening the file.
A file pointer is a pointer to this structure of the file.
File pointer is needed for the communication between the file and program .
A file pointer can be created as follow:
FILE *ptr;
ptr = fopen("filename.ext", "mode");
Link :- Click here to get the program to open a file in read and right mode.
File opening modes in C.
C offers the programmers to select a mode for opening a file.
Following modes are primarily used in C file I/O
"r" --> open for reading
"rb" --> open for reading in binary
If the file does not exist while reading fopen return null.
Type of Files
These are two types of files:
- Text files (.txt, .c)
- binary files(.jpg, .dat)
Reading a file
A file can be opened for reading as follows:
FILE *ptr;
ptr = fopen("Deepak.txt","r");
int num;
Let us assume that "Deepak.txt" Contains an integer We can read that integer using:
fscanf(ptr, "%d", &num); ==> fscanf is file counter part of Scanf
Link :- Click here to get the program of reading the file.
This will read an integer from file in num variable.
Quick Quiz: Modify the program above to check whether the file exists or not before opening the file.
Link:- Click here to get the solution of the quick quiz.
Closing the file :-
It is very important to close the file after read or write. this is achieved using fclose as follows :
fclose(ptr);
This will tell the compiler that we are done working with this file and the associated resources could be freed.
Writing to a file:-
we can write to a file in a very similar manner like we read the file
FILE *ptr;
fptr = fopen("Deepak.txt", "w");
int num = 432;
fprintf(fptr, "%d", num);
fclose(fptr);
link :- Click here to get the program to write in a file.
fgetc() and fputc()
fgets and fputc are used to read and write a character from/to a file
fgetc(ptr) => used to read a character from file
fputc('c', ptr); => used to write character c to the file.
Link:- Click here to get the program of fgetc and fputc.
EOF : End of File
fgtec returns EOF when all the character from a file has been read so we can write a check to detect the file .
Link:- Click here to check the program of implementation of EOF .
When all the content of the file has been read break the loop.
Practice set:-
1. write a program to read three integer from a file.
Link :- click here to get the solution of the above practice problem.
2. Write a program to generate multiplication table of a given number in text format make sure the file is readable and well formatted
Link:- Click here to get the solution of above practice problem.
3. Write a program to read a text file character by character and write it context twice in a separate file
Link :- Click here to get the solution of the above practice problem.
4. write a program to modify a file containing an integer to double its value.
Link:- Click here to get the solution of the above practice problem.
Project 2: snake, water, gun:-
snake, water, gun or rock, paper, scissors is a game most of us played during the school time.
Write a c program capable of playing this game with you
your program should be able to print the result after you choose snake /water or gun.
Link:- Click here to get the source code of the above game project.
Dynamic memory Allocation.
C is a language with some fixed rules of programming For example:- changing in size of array not allowed
Dynamic memory allocation:-
Dynamic memory allocation is a way to allocate memory to a data structure during the runtime We can use DMA function available in C to allocate and free memory during runtime.
Function for DMA in C
following function are available in C to perform dynamic memory allocation .
- malloc()
- calloc()
- free()
- recall()
Malloc() Function:-
malloc stands for memory allocation. It takes number of bytes to be allocated as an input and returns a pointer of type void
Link:- Click here to check the program of implementation of malloc.
The expression returns a null pointer if the memory cannot be allocated
calloc() Function:-
calloc stands for continuous allocation It initializes each memory block with a default value of 0.
Link:- Click here to check the implementation of calloc.
If the space is not sufficient , memory allocation is failed and a null pointer is returned.
Quick Quiz :- Write a program to create an array of size n using calloc where n is an integer entered by the user.
Link:- Click here to get the solution of above Quick quiz.
free() Function :-
we can use free() Function to de allocate the memory.
The memory allocated using calloc/malloc is not de allocated automatically.
syntax :-
free(ptr); => Memory of ptr is released.
Realloc() Function :-
sometimes the dynamically allocated memory is insufficient or more than required.
realloc is used to allocate memory of new size using the previous pointer and size
Synatx:-
ptr = realloc(ptr, newsize);
ptr = realloc(ptr, 3*sizeof(int));
ptr now points to this new block of memory capable of storing 3 integers.
Link:- Click here to check the implementation of realloc function in the program.
Practice set :
1. write a program to dynamically create an array of size 6 capable of storing 6 integers.
Link:- Click here to get the solution of the above practice problem.
2. use the array in problem 1 to store 6 integer entered by user
Link:- Click here to get the solution of the above practice problem.
3. solve problem 1 using calloc()
Link:- Click here to get the solution of above practice problem.
4. create an array dynamically capable of storing 5 integer . Now use realloc so that it can know store 10 integers.
Link:- Click here to get the solution of the above practice problem.
5. create an array of multiplication table of 7 up to 10(7x10 = 70). use realloc to make it store 15 number (from 7x1 to 7x15)
Link:- Click here to get the solution of above practice problem.
6. Attempt problem 4 using calloc()
Link:- Click here to get the solution of the above practice problem.
************* This is complete explanation of C language hope u like it ******************
So clear n informative completely mind boggling
ReplyDelete